uni-app 开发一个个税计算器。页面包含:计算功能,个税扣税规则。
以下是使用 Uni-app 开发一个简单个税计算器的详细步骤和代码示例。该计算器页面将包含计算功能以及个税扣税规则展示。
1. 创建 Uni-app 项目
首先,确保你已经安装了 HBuilderX 开发工具。打开 HBuilderX,选择 “文件” -> “新建” -> “项目”,选择 “uni-app” 模板,填写项目名称和保存路径,然后点击 “创建”。
2. 编写页面代码
<template>
<view class="container">
<!-- 标题 -->
<view class="title">个税计算器</view>
<!-- 输入框 -->
<view class="input-group">
<label>应纳税所得额(元):</label>
<input v-model="taxableIncome" type="number" placeholder="请输入应纳税所得额" />
</view>
<!-- 计算按钮 -->
<button @click="calculateTax">计算</button>
<!-- 计算结果展示 -->
<view v-if="taxAmount!== null" class="result">
<text>应缴纳个人所得税:{{ taxAmount.toFixed(2) }} 元</text>
</view>
<!-- 个税扣税规则 -->
<view class="rule">
<text>个税扣税规则:</text>
<ul>
<li>不超过 36000 元的部分,税率 3%,速算扣除数 0 元</li>
<li>超过 36000 元至 144000 元的部分,税率 10%,速算扣除数 2520 元</li>
<li>超过 144000 元至 300000 元的部分,税率 20%,速算扣除数 16920 元</li>
<li>超过 300000 元至 420000 元的部分,税率 25%,速算扣除数 31920 元</li>
<li>超过 420000 元至 660000 元的部分,税率 30%,速算扣除数 52920 元</li>
<li>超过 660000 元至 960000 元的部分,税率 35%,速算扣除数 85920 元</li>
<li>超过 960000 元的部分,税率 45%,速算扣除数 181920 元</li>
</ul>
</view>
</view>
</template>
<script>
export default {
data() {
return {
taxableIncome: '', // 应纳税所得额
taxAmount: null // 应缴纳个人所得税金额
};
},
methods: {
calculateTax() {
// 将输入的应纳税所得额转换为数字
const income = parseFloat(this.taxableIncome);
if (isNaN(income) || income < 0) {
uni.showToast({
title: '请输入有效的应纳税所得额',
icon: 'none'
});
this.taxAmount = null;
return;
}
let tax = 0;
if (income <= 36000) {
tax = income * 0.03;
} else if (income <= 144000) {
tax = income * 0.1 - 2520;
} else if (income <= 300000) {
tax = income * 0.2 - 16920;
} else if (income <= 420000) {
tax = income * 0.25 - 31920;
} else if (income <= 660000) {
tax = income * 0.3 - 52920;
} else if (income <= 960000) {
tax = income * 0.35 - 85920;
} else {
tax = income * 0.45 - 181920;
}
this.taxAmount = tax;
}
}
};
</script>
<style scoped>
.container {
padding: 20px;
}
.title {
font-size: 24px;
font-weight: bold;
text-align: center;
margin-bottom: 20px;
}
.input-group {
margin-bottom: 20px;
}
.input-group label {
display: block;
margin-bottom: 5px;
}
.input-group input {
width: 100%;
height: 40px;
border: 1px solid #ccc;
border-radius: 5px;
padding: 0 10px;
}
button {
width: 100%;
height: 40px;
background-color: #007aff;
color: #fff;
border: none;
border-radius: 5px;
font-size: 16px;
}
.result {
margin-top: 20px;
text-align: center;
font-size: 18px;
}
.rule {
margin-top: 20px;
}
.rule ul {
list-style-type: none;
padding: 0;
}
.rule li {
margin-bottom: 5px;
}
</style>
3. 代码解释
模板部分 (
<template>
):包含一个标题、输入框、计算按钮、计算结果展示区域和个税扣税规则展示区域。 使用 v-model
指令将输入框的值绑定到taxableIncome
数据属性。点击计算按钮时,调用 calculateTax
方法进行个税计算。脚本部分 (
<script>
):data
中定义了 taxableIncome
和taxAmount
两个数据属性。calculateTax
方法用于计算个税。首先检查输入的应纳税所得额是否有效,如果无效则弹出提示框;然后根据不同的税率区间计算应缴纳的个人所得税。 样式部分 (
<style>
):使用 scoped
属性确保样式只作用于当前组件。对页面元素进行了简单的样式设置,使其布局更加美观。
4. 运行项目
在 HBuilderX 中,选择要运行的平台(如微信小程序、H5 等),然后点击运行按钮即可在相应平台上查看和测试个税计算器。
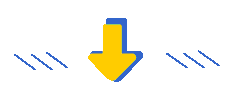
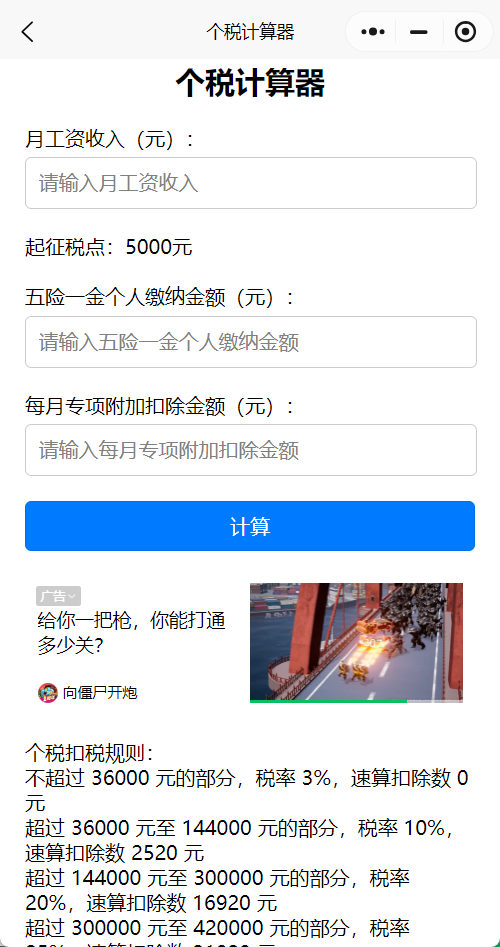
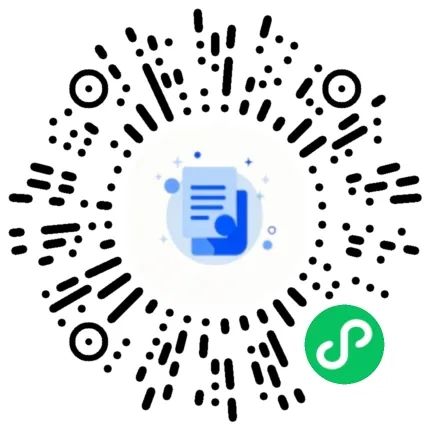